
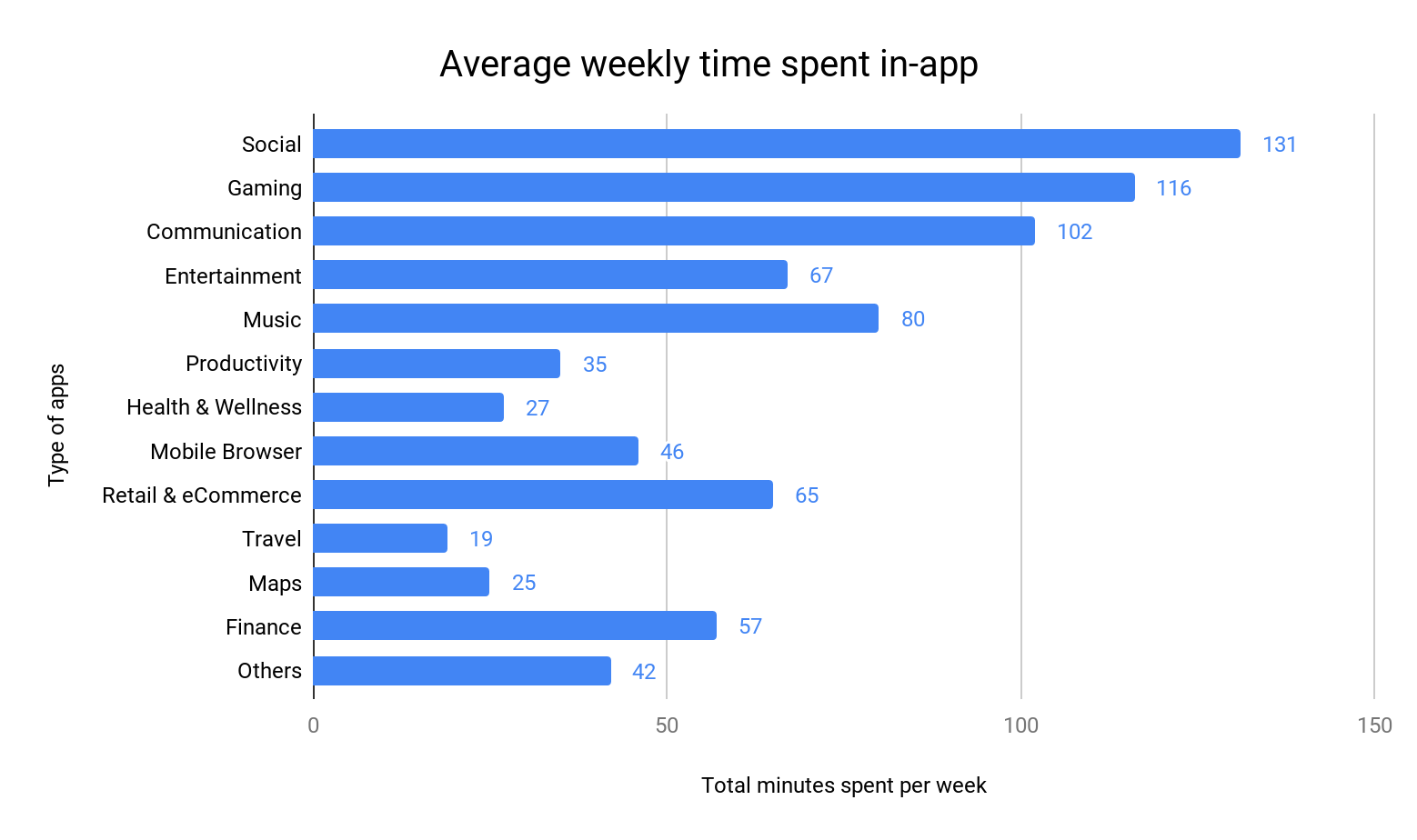
Print( "Element is present at index " + str(result))Įlement is present at index 2 Time complexity of Binary Search Result = BinarySearch(arr, k, 0, len(arr) - 1) Return BinarySearch(arr, k, mid + 1, high) Return BinarySearch(arr, k, low, mid - 1) Mid = low + (high - low) // 2 if arr = k:
#Average time for sequential search code#
Python Code for Binary Search (Iterative Method) We will repeat the same steps until the low pointer meets the high pointer and we find the desired element. If the element to be searched is lower than the mid element, we will set the high pointer to the "mid-1" element and run the algorithm again. If the element to be searched is greater than the mid, we will set the low pointer to the "mid+1" element and run the algorithm again. We will compare the mid element with the element to be searched and if it matches, we will return the mid element. Now, we will find the middle element of the array using the algorithm and set the mid pointer to it. Now, we will set two pointers pointing the low to the lowest position in the array and high to the highest position in the array. Return BinarySearch(array, k, low, mid - 1)Ĭonsider the following array on which the search is performed. Return BinarySearch(array, k, mid + 1, high) else // k is on the right side Return mid else if k > array // k is on the right side Algorithm for Binary Search (Iterative Method)īinarySearch(array, k, low, high) if low > high The steps of the process are general for both the methods, the difference is only found in the function calling. There are two methods by which we can run the binary search algorithm i.e, iterative method or recursive method. If the list is not sorted, then the algorithm first sorts the elements using the sorting algorithm and then runs the binary search function to find the desired output. The most important thing to note about binary search is that it works only on sorted lists of elements. Binary search algorithms are fast and effective in comparison to linear search algorithms. The searching operations is less than 100 itemsīinary search is used with a similar concept, i.e to find the element from the list of elements.Used to find the desired element from the collection of data when the dataset is small.The running time complexity of the linear search algorithm is O(n) for N number of elements in the list as the algorithm has to travel through each and every element to find the desired element. Print( "Element found at index: ", result)Įlement found at index: 3 Time Complexity of Linear Search If the element is found, we will return the index of that element else, we will return 'element not found'. If the match is not found, we will jump to the next element of the array and compare it with the element to be searched i.e ‘a’. We will start with the first element of the array, compare the first element with the element to be found. Now we have to find element a = 1 in the array given below. Example:Ĭonsider the below array of elements. Therefore, it is just a simple searching algorithm. If the element is not found in the list, the algorithm traverses the whole list and return “element not found”. The algorithm begins from the first element of the list, starts checking every element until the expected element is found. Linear search is also known as a sequential searching algorithm to find the element within the collection of data.
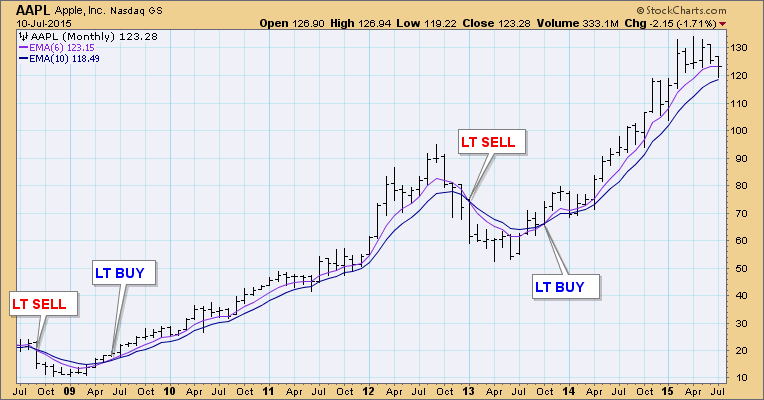
In this article, we will learn linear search and binary search in detail with algorithms, examples, and python code. There are many types of searching algorithms possible like linear search, binary search, jump search, exponential search, Fibonacci search, etc. And hence it is always said that the difference between the fast application and slower application is often decided by the searching algorithm used by the application. The searching algorithm is always considered to be the fundamental procedure of computing. Therefore, the searching algorithm is the set of procedures used to locate the specific data from the collection of data. The system jumps into its memory, processes the search of data using the searching algorithm technique, and returns the data that the user requires. When the data is stored in it and after a certain amount of time the same data is to be retrieved by the user, the computer uses the searching algorithm to find the data. The same thing happens with the computer system. There is not even a single day when we don’t want to find something in our daily life. So, let's get started! What is a searching algorithm? Lastly, we will understand the time complexity and application of the searching algorithm. We will learn their algorithm along with the python code and examples of the searching algorithms in detail. In this article, we will study what searching algorithms are and the types of searching algorithms i.e linear search and binary search in detail.
